Introduction
how to create a stunning Glassmorphism login form using HTML, CSS, and JavaScript. Follow this step-by-step guide to achieve a modern design.
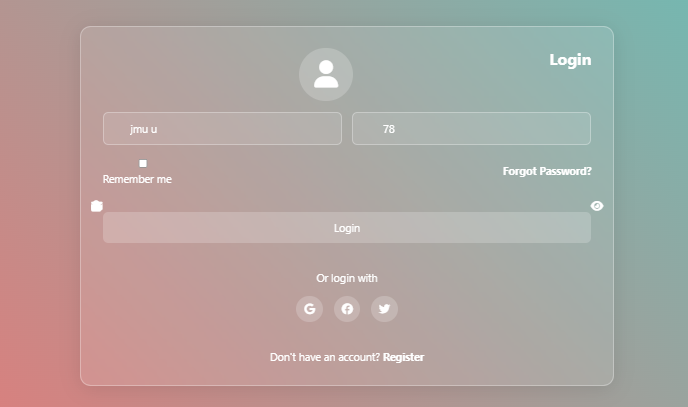
Prerequisites
- Basic knowledge of HTML, CSS, and JavaScript.
- A text editor like VS Code.
- A modern web browser for testing.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Glassmorphism Login Form</title>
<link rel="stylesheet" href="styles.css">
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/6.5.1/css/all.min.css">
<style>
* {
margin: 0;
padding: 0;
box-sizing: border-box;
font-family: 'Segoe UI', sans-serif;
}
.container {
min-height: 100vh;
display: flex;
justify-content: center;
align-items: center;
background: linear-gradient(45deg, #ff6b6b, #4ecdc4);
}
.login-card {
background: rgba(255, 255, 255, 0.1);
backdrop-filter: blur(10px);
border: 1px solid rgba(255, 255, 255, 0.3);
border-radius: 16px;
padding: 2rem;
width: 100%;
max-width: 800px;
box-shadow: 0 4px 30px rgba(0, 0, 0, 0.1);
display: flex;
flex-wrap: wrap;
}
.avatar {
width: 80px;
height: 80px;
background: rgba(255, 255, 255, 0.2);
border-radius: 50%;
margin: 0 auto 1rem;
display: flex;
align-items: center;
justify-content: center;
}
.avatar i {
font-size: 40px;
color: white;
}
h2 {
color: white;
text-align: center;
margin-bottom: 2rem;
}
.input-group {
flex: 1 1 calc(50% - 1rem);
min-width: 250px;
}
.input-group i {
position: absolute;
left: 15px;
top: 50%;
transform: translateY(-50%);
color: white;
}
.input-group .toggle-password {
left: auto;
right: 15px;
cursor: pointer;
}
input {
width: 100%;
padding: 12px 40px;
background: rgba(255, 255, 255, 0.1);
border: 1px solid rgba(255, 255, 255, 0.3);
border-radius: 8px;
color: white;
font-size: 16px;
transition: all 0.3s ease;
}
input::placeholder {
color: rgba(255, 255, 255, 0.7);
}
input:focus {
outline: none;
border-color: rgba(255, 255, 255, 0.6);
}
.remember-forgot {
flex: 1 1 100%;
display: flex;
justify-content: space-between;
align-items: center;
margin-bottom: 1.5rem;
color: white;
}
button {
flex: 1 1 100%;
width: 100%;
padding: 12px;
background: rgba(255, 255, 255, 0.2);
border: none;
border-radius: 8px;
color: white;
font-size: 16px;
cursor: pointer;
transition: all 0.3s ease;
}
button:hover {
background: rgba(255, 255, 255, 0.3);
}
.social-login {
flex: 1 1 100%;
text-align: center;
margin-top: 1.5rem;
color: white;
}
.social-icons {
display: flex;
justify-content: center;
gap: 1rem;
margin-top: 1rem;
}
.social-icons a {
width: 40px;
height: 40px;
background: rgba(255, 255, 255, 0.2);
border-radius: 50%;
display: flex;
align-items: center;
justify-content: center;
color: white;
text-decoration: none;
transition: all 0.3s ease;
}
.social-icons a:hover {
background: rgba(255, 255, 255, 0.3);
transform: translateY(-2px);
}
.register-link {
flex: 1 1 100%;
text-align: center;
margin-top: 1.5rem;
color: white;
}
.register-link a, .forgot-link {
color: white;
text-decoration: none;
font-weight: bold;
}
#loginForm {
display: flex;
flex-wrap: wrap;
gap: 1rem;
}
@media (max-width: 768px) {
.login-card {
margin: 1rem;
padding: 1.5rem;
max-width: 400px;
}
.input-group {
flex: 1 1 100%;
}
}
<style/>
</head>
<body>
<div class="container">
<div class="login-card">
<div class="avatar">
<i class="fas fa-user"></i>
</div>
<h2>Login</h2>
<form id="loginForm">
<div class="input-group">
<i class="fas fa-envelope"></i>
<input type="email" id="email" placeholder="Email" required>
</div>
<div class="input-group">
<i class="fas fa-lock"></i>
<input type="password" id="password" placeholder="Password" required>
<i class="fas fa-eye-slash toggle-password"></i>
</div>
<div class="remember-forgot">
<label>
<input type="checkbox" id="remember">
Remember me
</label>
<a href="#" class="forgot-link">Forgot Password?</a>
</div>
<button type="submit">Login</button>
<div class="social-login">
<p>Or login with</p>
<div class="social-icons">
<a href="#"><i class="fab fa-google"></i></a>
<a href="#"><i class="fab fa-facebook"></i></a>
<a href="#"><i class="fab fa-twitter"></i></a>
</div>
</div>
<p class="register-link">
Don't have an account? <a href="#">Register</a>
</p>
</form>
</div>
</div>
<script src="script.js"></script>
<script>
document.addEventListener('DOMContentLoaded', () => {
const loginForm = document.getElementById('loginForm');
const togglePassword = document.querySelector('.toggle-password');
const passwordInput = document.getElementById('password');
// Toggle password visibility
togglePassword.addEventListener('click', () => {
const type = passwordInput.getAttribute('type') === 'password' ? 'text' : 'password';
passwordInput.setAttribute('type', type);
togglePassword.classList.toggle('fa-eye');
togglePassword.classList.toggle('fa-eye-slash');
});
// Form submission
loginForm.addEventListener('submit', (e) => {
e.preventDefault();
const email = document.getElementById('email').value;
const password = passwordInput.value;
// Basic email validation
const emailRegex = /^[^\s@]+@[^\s@]+\.[^\s@]+$/;
if (!emailRegex.test(email)) {
alert('Please enter a valid email address');
return;
}
// Basic password validation
if (password.length < 6) {
alert('Password must be at least 6 characters long');
return;
}
// Here you would typically send the data to a server
console.log('Form submitted:', { email, password });
});
// Add hover effects to social icons
const socialIcons = document.querySelectorAll('.social-icons a');
socialIcons.forEach(icon => {
icon.addEventListener('mouseenter', () => {
icon.style.transform = 'translateY(-5px)';
});
icon.addEventListener('mouseleave', () => {
icon.style.transform = 'translateY(0)';
});
});
});
<script/>
</body>
</html>
HTML Structure for Glassmorphism Login Form
- Container: Wraps the entire form.
- Form Card: The glass-effect form where input fields and buttons reside.
- Input Fields: For Username and Password.
- Buttons: Login and optional Register button.
- Links: Forgot password or signup options.
SEO Tip: Use semantic HTML elements and descriptive alt
attributes for images.
CSS Styling for Glassmorphism Effect
- Background:
- Use vibrant gradients or high-resolution images.
- Apply a blur effect to enhance the glass effect.
- Form Card:
- Background:
rgba(255, 255, 255, 0.1)
for transparency. - Backdrop Filter:
backdrop-filter: blur(10px);
for the frosted effect. - Border:
1px solid rgba(255, 255, 255, 0.3);
for subtle borders. - Box-Shadow:
0 4px 30px rgba(0, 0, 0, 0.1);
for depth.
- Background:
- Input Fields and Buttons:
- Transparent background with soft borders.
- Smooth hover transitions for a modern feel.
- Typography:
- Use clean and readable fonts.
- Ensure high contrast for accessibility.
SEO Tip: Use CSS variables for easy customization and better code maintenance.
JavaScript Functionalities
- Form Validation:
- Check for empty fields and incorrect formats (like email).
- Display dynamic error messages.
- Password Toggle:
- Option to show/hide passwords for better usability.
- Interactive Buttons:
- Add hover effects and animations for better engagement.
- Form Submission (Optional):
- Handle form data dynamically using JavaScript.
SEO Tip: Ensure fast loading of scripts and minimize unnecessary functions.
Responsive Design Tips
- Use percentage-based widths and media queries for mobile responsiveness.
- Adjust padding and margins for different screen sizes.
- Test the design across various devices.
SEO Tip: Mobile-friendly designs rank higher on search engines.
Additional Enhancements
- Include subtle animations for form elements.
- Add social media icons for login options.
- Use an avatar icon for personalization.
- Consider dark mode compatibility using CSS variables.
Common Mistakes to Avoid
- Overusing the blur effect which can hinder readability.
- Ignoring accessibility standards like proper contrast and ARIA labels.
- Not optimizing images and assets, which can slow down page loading.
Conclusion
Creating a Glassmorphism login form enhances the visual appeal and user engagement of your website. By following the steps above and implementing SEO best practices, you can ensure a high-performing and attractive design.
SEO Tip: Regularly update your website’s UI and optimize for speed to maintain high search rankings.
FAQs
Q1: What is Glassmorphism?
- Glassmorphism is a UI design trend that uses transparency, blur, and vivid backgrounds to create a glass-like effect.
Q2: Is Glassmorphism suitable for all types of websites?
- Yes, it can be adapted for various types of websites, including portfolios, login pages, and landing pages.
Q3: How to improve the accessibility of a Glassmorphism login form?
A common practice in web development is to utilize JavaScript for form validation. This is not just about checking if fields are empty but also about enhancing the user experience. For example, if a user enters an invalid email address, providing immediate feedback can help them correct it before submission, which ultimately leads to a smoother interaction.