This registration page includes:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Register</title>
<style>
* {
margin: 0;
padding: 0;
box-sizing: border-box;
font-family: 'Poppins', sans-serif;
}
body {
min-height: 100vh;
display: flex;
justify-content: center;
align-items: center;
transition: background 1.5s ease;
}
.container {
position: relative;
width: 400px;
background: rgba(255, 255, 255, 0.95);
padding: 40px;
border-radius: 15px;
box-shadow: 0 0 20px rgba(0, 0, 0, 0.2);
animation: fadeIn 1s ease-in;
}
@keyframes fadeIn {
from { opacity: 0; transform: translateY(-20px); }
to { opacity: 1; transform: translateY(0); }
}
h2 {
text-align: center;
color: #333;
margin-bottom: 30px;
font-size: 28px;
}
.input-box {
position: relative;
margin-bottom: 35px;
}
.input-box input {
width: 100%;
padding: 12px;
background: transparent;
border: none;
border-bottom: 2px solid #4203a9;
outline: none;
font-size: 16px;
color: #333;
transition: all 0.3s ease;
}
.input-box label {
position: absolute;
left: 0;
top: 12px;
color: #666;
transition: all 0.3s ease;
pointer-events: none;
}
.input-box input:focus ~ label,
.input-box input:valid ~ label {
top: -20px;
font-size: 12px;
color: #4203a9;
}
.input-box input:focus {
border-bottom-color: #90bafc;
}
.btn {
width: 100%;
padding: 12px;
background: #4203a9;
border: none;
border-radius: 25px;
color: white;
font-size: 16px;
cursor: pointer;
transition: all 0.4s ease;
}
.btn:hover {
background: #90bafc;
transform: translateY(-2px);
box-shadow: 0 5px 15px rgba(144, 186, 252, 0.4);
}
.login-link {
text-align: center;
margin-top: 20px;
color: #666;
}
.login-link a {
color: #4203a9;
text-decoration: none;
transition: all 0.3s ease;
}
.login-link a:hover {
color: #90bafc;
text-decoration: underline;
}
</style>
</head>
<body>
<div class="container">
<h2>Create Account</h2>
<form id="registerForm">
<div class="input-box">
<input type="text" id="username" required>
<label>Username</label>
</div>
<div class="input-box">
<input type="email" id="email" required>
<label>Email</label>
</div>
<div class="input-box">
<input type="password" id="password" required>
<label>Password</label>
</div>
<button type="submit" class="btn">Register</button>
<div class="login-link">
Already have an account? <a href="#">Login</a>
</div>
</form>
</div>
<script>
// Background color changing functionality
const colors = [
'linear-gradient(135deg, #4203a9, #90bafc)',
'linear-gradient(135deg, #ff6b6b, #ffb88c)',
'linear-gradient(135deg, #2ecc71, #7ed56f)',
'linear-gradient(135deg, #3498db, #a1c4fd)',
'linear-gradient(135deg, #9b59b6, #e056fd)'
];
let currentIndex = 0;
function changeBackground() {
document.body.style.background = colors[currentIndex];
currentIndex = (currentIndex + 1) % colors.length;
}
// Initial background
changeBackground();
// Change background every 5 seconds
setInterval(changeBackground, 5000);
// Form handling
document.getElementById('registerForm').addEventListener('submit', function(e) {
e.preventDefault();
const username = document.getElementById('username').value;
const email = document.getElementById('email').value;
const password = document.getElementById('password').value;
if(username.length < 3) {
alert('Username must be at least 3 characters long');
return;
}
if(!email.includes('@') || !email.includes('.')) {
alert('Please enter a valid email address');
return;
}
if(password.length < 6) {
alert('Password must be at least 6 characters long');
return;
}
console.log('Registration attempt:', {
username,
email,
password
});
alert('Registration successful! (This is a demo)');
this.reset();
});
</script>
</body>
</html>
- Color Scheme:
- Primary color: #4203a9 (deep purple)
- Secondary color: #90bafc (light blue)
- Clean white background with subtle transparency
- Animations:
- Fade-in effect when the page loads
- Smooth label transitions when focusing inputs
- Button hover animation with lift and shadow effects
- Color transitions on hover states
- Features:
- Floating label effect for inputs
- Responsive design
- Gradient background
- Modern shadow effects
- Basic form validation
- Smooth transitions for all interactive elements
- Hover Effects:
- Button lifts and changes color with shadow
- Link color changes with underline effect
- Input border color changes on focus
- https://thinkcrft.com/create-a-stunning-glacier-login-form-with-snowfall-animation/JavaScript:
- Form submission handling
- Basic input validation
- Console logging of form data
- Success message (demo version)
Create Account
To use this:
- Save it as an HTML file
- Open it in a web browser
- The form will validate inputs and show a success message
- In a real application, you'd replace the console.log with an API call to your backend
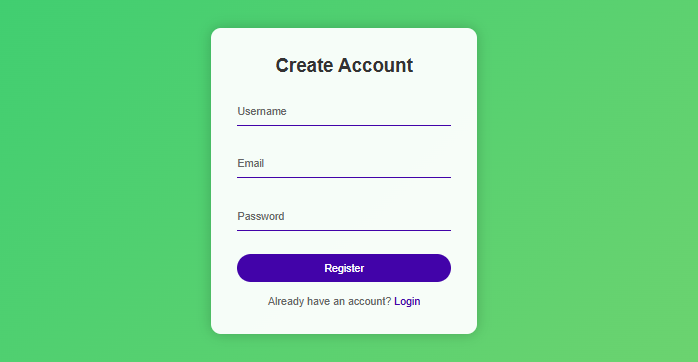
The design is clean, modern, and user-friendly with a focus on smooth interactions and visual feedback. You can customize the colors, sizes, and animations by modifying the CSS variables and values to match your preferences!